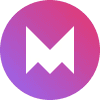
Motion values overview
Motion values track the state and velocity of animated values.
They are composable, signal-like values that are performant because Motion can render them with its optimised DOM renderer.
Usually, these are created automatically by motion
components. But for advanced use cases, it's possible to create them manually.
By manually creating motion values you can:
Set and get their state.
Pass to multiple components to synchronise motion across them.
Chain
MotionValue
s via theuseTransform
hook.Update visual properties without triggering React's render cycle.
Subscribe to updates.
Usage
Motion values can be created with the useMotionValue
hook. The string or number passed to useMotionValue
will act as its initial state.
Motion values can be passed to a motion
component via style
:
Or for SVG attributes, via the attribute prop itself:
It's possible to pass the same motion value to multiple components.
Motion values can be updated with the set
method.
Changes to the motion value will update the DOM without triggering a React re-render. Motion values can be updated multiple times but renders will be batched to the next animation frame.
A motion value can hold any string or number. We can read it with the get
method.
Motion values containing a number can return a velocity via the getVelocity
method. This returns the velocity as calculated per second to account for variations in frame rate across devices.
For strings and colors, getVelocity
will always return 0
.
Events
Listeners can be added to motion values via the on
method or the useMotionValueEvent
hook.
Available events are "change"
, "animationStart"
, "animationComplete"
"animationCancel"
.
Composition
Beyond useMotionValue
, Motion provides a number of hooks for creating and composing motion values, like useSpring
and useTransform
.
For example, with useTransform
we can take the latest state of one or more motion values and create a new motion value with the result.
useSpring
can make a motion value that's attached to another via a spring.
These motion values can then go on to be passed to motion
components, or composed with more hooks like useVelocity
.
API
get()
Returns the latest state of the motion value.
getVelocity()
Returns the latest velocity of the motion value. Returns 0
if the value is non-numerical.
set()
Sets the motion value to a new state.
jump()
Jumps the motion value to a new state in a way that breaks continuity from previous values:
Resets
velocity
to0
.Ends active animations.
Ignores attached effects (for instance
useSpring
's spring).
isAnimating()
Returns true
if the value is currently animating.
stop()
Stop the active animation.
on()
Subscribe to motion value events. Available events are:
change
animationStart
animationCancel
animationComplete
It returns a function that, when called, will unsubscribe the listener.
When calling on
inside a React component, it should be wrapped with a useEffect
hook, or instead use the useMotionValueEvent
hook.
destroy()
Destroy and clean up subscribers to this motion value.
This is normally handled automatically, so this method is only necessary if you've manually created a motion value outside the React render cycle using the vanilla motionValue
hook.
Motion is open source. Sponsorships keep the project sustainable.
Every sponsor receives access to our private Discord, and an exclusive mobile and desktop wallpaper pack.